Customizing the Initial Offer Incentive (IOI)
If you are trying to offer your customers an Initial Offer Incentive, you may be able to use our feature in your Ordergroove Admin. However, if you want to customize your customer experience even further, recommend you use a Shopify Script.
Shopify Scripts are small pieces of code that let you create unique experiences for your customers in their cart and at checkout. Find out more in Shopify's Help Center articles.
Getting Started
Our script below is a starting point that allows you to give customers a percent or dollar amount off their subscription purchase. To get started, install the Shopify Script Editor app if you don't already have it.
Create a new script for "Line Items" and begin with a blank template:
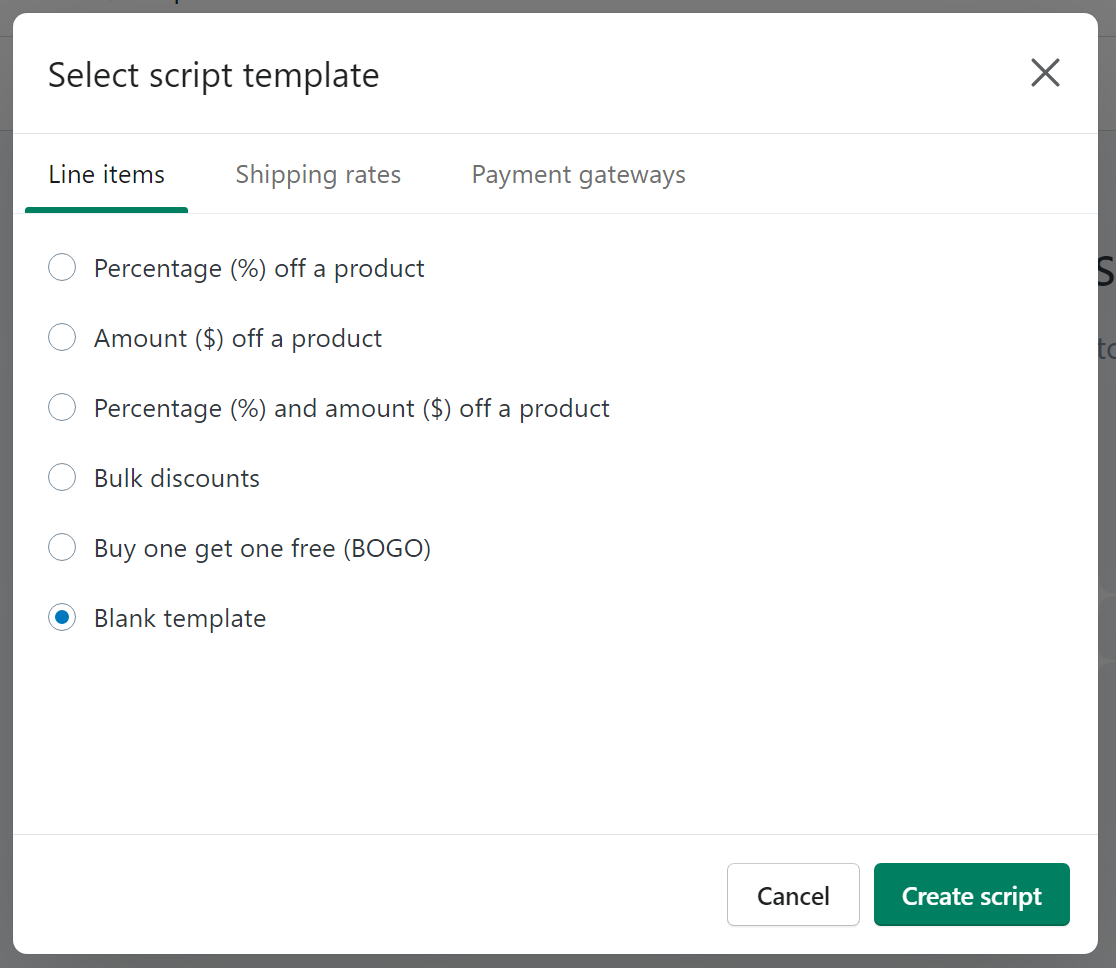
Once you have that, you can add the following code. This script will give a customer a discount on the subscription item only for whatever percentage you set. You can change the discount_amount and the discount_message to whatever you'd like. If you'd like to change this further, we recommend using Shopify's documentation on their Script Editor.
DISCOUNTS = [
{
discount_type: :percent,
discount_amount: 25,
discount_message: '25% off subscription products!'
}
]
class DiscountApplicator
def initialize(discount_type, discount_amount, discount_message)
@discount_type = discount_type
@discount_message = discount_message
@discount_amount = if discount_type == :percent
1 - (discount_amount * 0.01)
else
Money.new(cents: 100) * discount_amount
end
end
def apply(line_item)
new_line_price = if @discount_type == :percent
line_item.line_price * @discount_amount
else
[line_item.line_price - (@discount_amount * line_item.quantity), Money.zero].max
end
line_item.change_line_price(new_line_price, message: @discount_message)
end
end
class OrdergrooveDiscountCampaign
def initialize(discounts)
@discounts = discounts
end
def subscription(line_item)
!line_item.selling_plan_id.nil?
end
def run(cart)
@discounts.each do |discount|
discount_applicator = DiscountApplicator.new(
discount[:discount_type],
discount[:discount_amount],
discount[:discount_message]
)
cart.line_items.each do |line_item|
if subscription(line_item)
discount_applicator.apply(line_item)
end
end
end
end
end
CAMPAIGNS = [
OrdergrooveDiscountCampaign.new(DISCOUNTS),
]
CAMPAIGNS.each do |campaign|
campaign.run(Input.cart)
end
Output.cart = Input.cart
Once you copied the code in, click on the Save and Publish button to complete it.
Further Customizations
For further customizations to the above script, you can reference Shopify's excellent documentation on the Shopify Help Center. You'll find great examples of popular scripts and more that you can adapt and use with your subscription program.
Updated about 1 year ago